AJAX
AJAX簡介
- 全名為 Asynchronous JavaScript and XML,非同步JS與 XML ,透過 AJAX 可以在瀏覽器向伺服器發送請求,優勢在於,無須更新頁面得到資料。
- AJAX 不是新的程式語言,是一種將現有標準組合再一起使用的新方式。
XML介紹
- XML 可延伸標記式語言
- XML 被設計用來傳輸與儲存資料
- XML 與 HTML 相似,不同的是 HTML 中都是預先定義好的標籤,而 XML 沒有預定標籤,全部都是自定義來顯示資料。
//一般資料
name = "Alan" ; age = 18 ; gender = "男" ;
//XML
<student>
<name> Alan </name>
<age> 18 </age>
<gender> 男 <gender>
</student>
- 現今大多被JSON取代。
{"name" : "Alan", "age" : 18, "gender" : "男"}
Node.js 安裝
- 安裝好後,在 cmd 輸入 node -v,會出現版本
Express 框架介紹與基本使用
- AJAX 需要向 Server 發送請求
- 建立資料夾
- VScode 開啟進入 Terminal
- 輸入 npm init - - yes (npm的初始化)
- 安裝 express ⇒ 輸入 npm i express
- 建立一個 basic_use.js 的檔案
- basic_use.js 輸入以下
//1.導入express
const express = require('express');
//2.建立實體
const app = express();
//3.建立url
app.get('/', (request, response)=>{
response.send('Hello');
});
//4.監聽Port
app.listen(8000, ()=>{
console.log("Server Start, 8000 port listen")
});
7. 進入 basic_use.js 的路徑並開始 Terminal
8. 輸入 node basic_use.js
9. 已啟動 Server ,網址輸入127.0.0.1:8000
AJAX 事前準備
- 前端準備 1個 1-GET.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX GET 請求</title>
<style>
#result{
width: 200px;
height: 100px;
border: solid 1px #90b;
}
</style>
</head>
<body>
<button>submit</button>
<div id="result"></div>
</body>
</html>
- 後端準備 1個 server.js
const express = require('express');
const app = express();
app.get('/server', (request, response)=>{
//允許跨域
response.setHeader('Access-Control-Allow-Origin','*');
response.send('Hello');
});
app.listen(8000, ()=>{
console.log("Server Start, 8000 port listen")
});
- 啟動 server.js
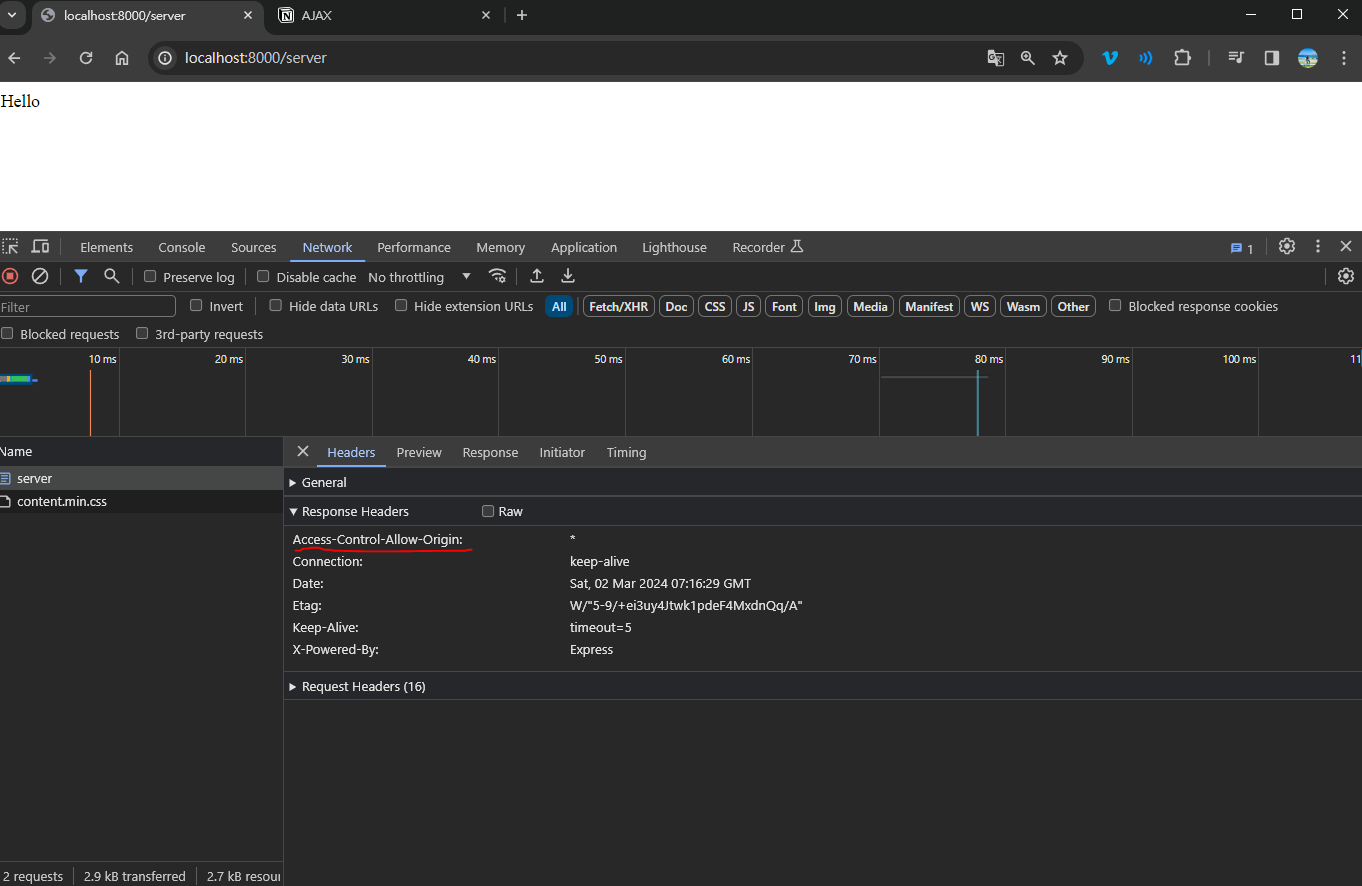
AJAX 請求的基本操作
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX GET 請求</title>
<style>
#result{
width: 200px;
height: 100px;
border: solid 1px #90b;
}
</style>
</head>
<body>
<button>submit</button>
<div id="result"></div>
<script>
const btn = document.getElementsByTagName('button')[0];
const result = document.getElementById("result");
btn.onclick = function(){
//1.創建實體
const xhr = new XMLHttpRequest();
//2.初始化
xhr.open('GET', 'http:/127.0.0.1:8000/server')
//3.發送
xhr.send();
//4.事件綁定
//readystate 是 xhr 的對象中屬性, 表示狀態 0 1 2 3 4
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
// 判斷回應碼 200 404 403 401 500
// 2xx 成功
if(xhr.status === 200 && xhr.status < 300){
// console.log(xhr.status);
// console.log(xhr.statusText);
// console.log(xhr.getAllResponseHeaders);
// console.log(xhr.response);
result.innerHTML = xhr.response;
}
else{
}
}
}
}
</script>
</body>
</html>
AJAX 設置參數
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX GET 請求</title>
<style>
#result{
width: 200px;
height: 100px;
border: solid 1px #90b;
}
</style>
</head>
<body>
<button>submit</button>
<div id="result"></div>
<script>
const btn = document.getElementsByTagName('button')[0];
const result = document.getElementById("result");
btn.onclick = function(){
//1.創建實體
const xhr = new XMLHttpRequest();
//2.初始化
xhr.open('GET', 'http:/127.0.0.1:8000/server?a=100&b=200&c=300')
//3.發送
xhr.send();
//4.事件綁定
//readystate 是 xhr 的對象中屬性, 表示狀態 0 1 2 3 4
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
// 判斷回應碼 200 404 403 401 500
// 2xx 成功
if(xhr.status === 200 && xhr.status < 300){
// console.log(xhr.status);
// console.log(xhr.statusText);
// console.log(xhr.getAllResponseHeaders);
// console.log(xhr.response);
result.innerHTML = xhr.response;
}
else{
}
}
}
}
</script>
</body>
</html>
AJAX 發送 POST 請求
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX POST 請求</title>
<style>
#result{
width: 200px;
height: 100px;
border: solid 1px #903;
}
</style>
</head>
<body>
<div id="result"></div>
<script>
const result = document.getElementById("result");
result.addEventListener("mouseover", function(){
const xhr = new XMLHttpRequest();
xhr.open('POST', 'http://127.0.0.1:8000/server');
xhr.send();
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
if(xhr.status>=200 && xhr.status<300){
result.innerHTML = xhr.response;
}
}
};
});
</script>
</body>
</html>
const express = require('express');
const app = express();
app.get('/server', (request, response)=>{
//允許跨域
response.setHeader('Access-Control-Allow-Origin','*');
response.send('Hello');
});
app.post('/server', (request, response)=>{
//允許跨域
response.setHeader('Access-Control-Allow-Origin','*');
response.send('Hello 123');
});
app.listen(8000, ()=>{
console.log("Server Start, 8000 port listen")
});
AJAX 發送 POST 參數
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX POST 請求</title>
<style>
#result{
width: 200px;
height: 100px;
border: solid 1px #903;
}
</style>
</head>
<body>
<div id="result"></div>
<script>
const result = document.getElementById("result");
result.addEventListener("mouseover", function(){
const xhr = new XMLHttpRequest();
xhr.open('POST', 'http://127.0.0.1:8000/server');
xhr.send('a=100&b=200&c=300');
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
if(xhr.status>=200 && xhr.status<300){
result.innerHTML = xhr.response;
}
}
};
});
</script>
</body>
</html>
AJAX RequestHeader 設定請求
AJAX 伺服器返回JSON資料
AJAX nodemon 自動啟動伺服器工具安裝
AJAX 請求超時與網路異常處理
AJAX 取消請求
AJAX 請求重複發送問題
AJAX JQuery 發送AJAX請求
AJAX JQuery 通用方法發送AJAX請求
Axios 發送 AJAX 請求
Axios 函數發送AJAX請求
使用 fetch 函數發送AJAX請求
同源政策 (Same-origin policy)
jsonp的實現原理
原生jsonp實作
JQuery發送jsonp請求